๋ฌธ์
https://leetcode.com/problems/unique-binary-search-trees-ii/
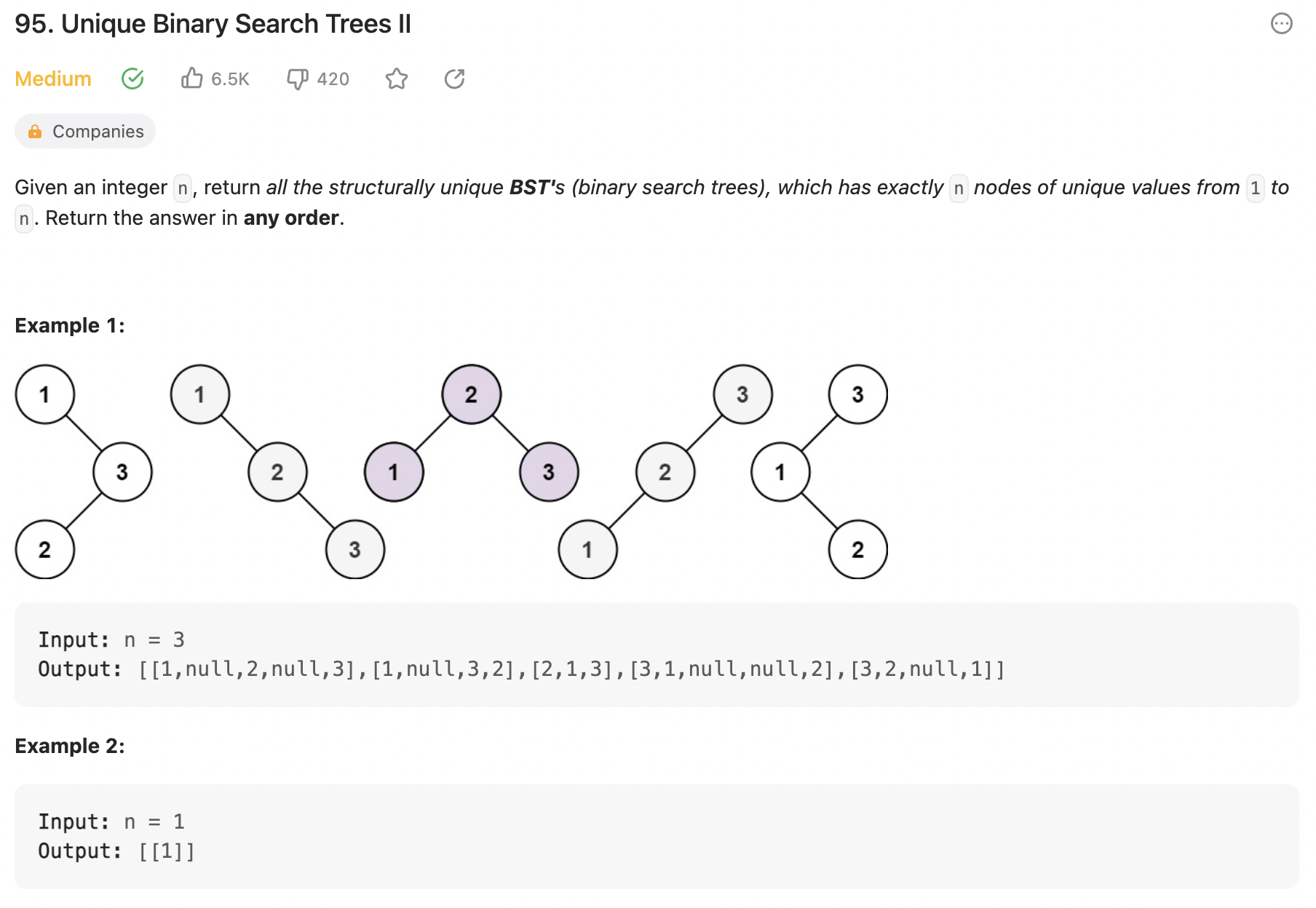
์ฃผ์ด์ง n์ ๋ํด, ๋ชจ๋ ๊ตฌ์กฐ์ ์ผ๋ก ์ ์ผํ BST(์ด์ง ๊ฒ์ ํธ๋ฆฌ)๋ฅผ ๋ฐํํ๋ผ. BST๋ 1๋ถํฐ n๊น์ง ๊ณ ์ ํ ๊ฐ์ ๊ฐ๋ n๊ฐ์ ๋ ธ๋๋ก ๊ตฌ์ฑ๋๋ค. ๋ต์ ๋ฐํํ๋๋ฐ์ ์์๋ ๋ฌด๊ดํ๋ค.
ํ์ด
DP์ ๋ฉ๋ชจ์ด์ ์ด์ ์ ์ด์ฉ - ๊ฐ์ฅ ์์ ํธ๋ฆฌ๋ ธ๋๋ถํฐ ๋ง๋ค์ด ์ด๋ฅผ ๊ธฐ๋กํด๋๊ณ , ๊ณ์ํด์ ํด๋น memo๋ฅผ ์ฌ์ฉํ๋ ๋ฐฉ์
์๊ฐ ๋ณต์ก๋: O(n^2), ๊ณต๊ฐ ๋ณต์ก๋: O(n^2)
1. JAVA
public class LeetCode_95_UniqueBinarySearchTrees2 {
public static class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode() {}
TreeNode(int val) { this.val = val; }
TreeNode(int val, TreeNode left, TreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}
public static class StartEnd {
int start;
int end;
StartEnd(int start, int end) {
this.start = start;
this.end = end;
}
}
// Start๋ถํฐ End๊น์ง์ ๊ฐ๋ฅํ ๋ชจ๋ ํธ๋ฆฌ๋
ธ๋๋ค์ ์ ์ฅํด๋์
public static Map<StartEnd, List<TreeNode>> memo = new HashMap<>();
public static List<TreeNode> treesStartToEnd(int start, int end) {
List<TreeNode> tn = new ArrayList<>();
if (start > end) {
tn.add(null);
return tn;
}
if (start == end) {
tn.add(new TreeNode(start));
return tn;
}
if (memo.containsKey(new StartEnd(start, end))) { // memoization
return memo.get(new StartEnd(start, end));
}
for (int i = start; i <= end; i++) {
List<TreeNode> left = treesStartToEnd(start, i - 1);
List<TreeNode> right = treesStartToEnd(i + 1, end);
for(TreeNode l: left) {
for(TreeNode r : right) {
TreeNode root = new TreeNode(i);
root.left = l;
root.right = r;
tn.add(root);
}
}
}
memo.put(new StartEnd(start, end), tn);
return tn;
}
public static List<TreeNode> generateTrees(int n) {
return treesStartToEnd(1, n);
}
}

2. Kotlin
class TreeNode(var val: Int) {
var left: TreeNode? = null
var right: TreeNode? = null
}
val memo: MutableMap<Pair<Int, Int>, MutableList<TreeNode?>> = mutableMapOf()
fun treesStartToEnd(start: Int, end: Int): MutableList<TreeNode?> {
val tn: MutableList<TreeNode?> = mutableListOf()
if (start > end) {
tn.add(null)
return tn
}
if (start == end) {
tn.add(TreeNode(start))
return tn
}
if (memo.contains((start to end))) {
return memo[(start to end)]!!
}
for (i in start..end) {
val left = treesStartToEnd(start, i - 1)
val right = treesStartToEnd(i + 1, end)
left.forEach { l ->
right.forEach { r ->
tn.add(
TreeNode(i).also {
it.right = r
it.left = l
}
)
}
}
}
memo[(start to end)] = tn
return tn
}
fun generateTrees(n: Int): List<TreeNode?> {
return treesStartToEnd(1, n)
}

https://github.com/jeongum/algorithm/blob/master/dp/LT_95_UniqueBinarySearchTrees2.kt
'๐ ์๊ณ ๋ฆฌ์ฆ > Dynamic Programming' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[LeetCode] 403. Frog Jump (ํด์, Java ํ์ด) (0) | 2023.08.28 |
---|---|
[์๊ณ ๋ฆฌ์ฆ] Dynamic Programming (0) | 2023.08.05 |
๋ฌธ์
https://leetcode.com/problems/unique-binary-search-trees-ii/
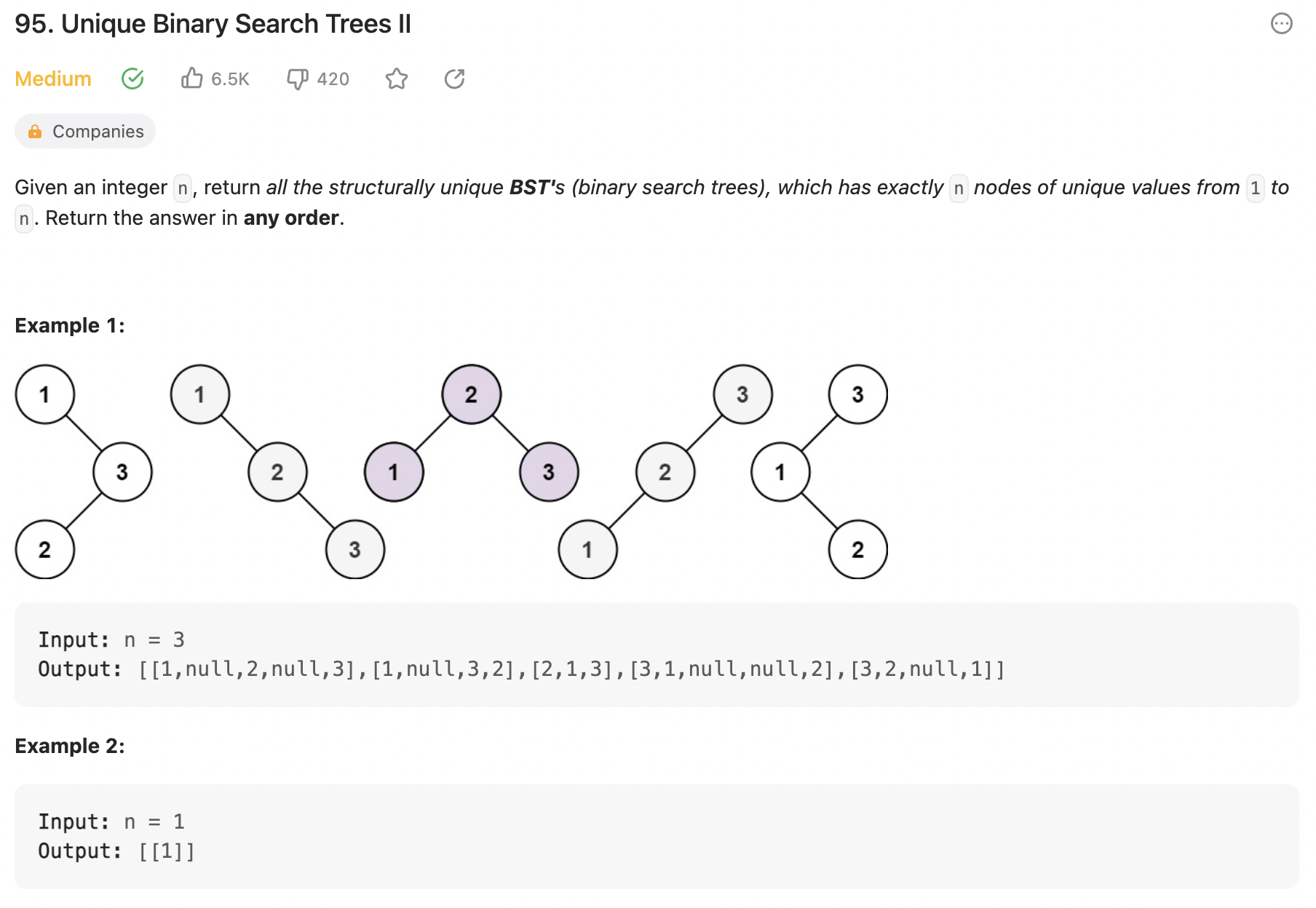
์ฃผ์ด์ง n์ ๋ํด, ๋ชจ๋ ๊ตฌ์กฐ์ ์ผ๋ก ์ ์ผํ BST(์ด์ง ๊ฒ์ ํธ๋ฆฌ)๋ฅผ ๋ฐํํ๋ผ. BST๋ 1๋ถํฐ n๊น์ง ๊ณ ์ ํ ๊ฐ์ ๊ฐ๋ n๊ฐ์ ๋ ธ๋๋ก ๊ตฌ์ฑ๋๋ค. ๋ต์ ๋ฐํํ๋๋ฐ์ ์์๋ ๋ฌด๊ดํ๋ค.
ํ์ด
DP์ ๋ฉ๋ชจ์ด์ ์ด์ ์ ์ด์ฉ - ๊ฐ์ฅ ์์ ํธ๋ฆฌ๋ ธ๋๋ถํฐ ๋ง๋ค์ด ์ด๋ฅผ ๊ธฐ๋กํด๋๊ณ , ๊ณ์ํด์ ํด๋น memo๋ฅผ ์ฌ์ฉํ๋ ๋ฐฉ์
์๊ฐ ๋ณต์ก๋: O(n^2), ๊ณต๊ฐ ๋ณต์ก๋: O(n^2)
1. JAVA
public class LeetCode_95_UniqueBinarySearchTrees2 {
public static class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode() {}
TreeNode(int val) { this.val = val; }
TreeNode(int val, TreeNode left, TreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}
public static class StartEnd {
int start;
int end;
StartEnd(int start, int end) {
this.start = start;
this.end = end;
}
}
// Start๋ถํฐ End๊น์ง์ ๊ฐ๋ฅํ ๋ชจ๋ ํธ๋ฆฌ๋
ธ๋๋ค์ ์ ์ฅํด๋์
public static Map<StartEnd, List<TreeNode>> memo = new HashMap<>();
public static List<TreeNode> treesStartToEnd(int start, int end) {
List<TreeNode> tn = new ArrayList<>();
if (start > end) {
tn.add(null);
return tn;
}
if (start == end) {
tn.add(new TreeNode(start));
return tn;
}
if (memo.containsKey(new StartEnd(start, end))) { // memoization
return memo.get(new StartEnd(start, end));
}
for (int i = start; i <= end; i++) {
List<TreeNode> left = treesStartToEnd(start, i - 1);
List<TreeNode> right = treesStartToEnd(i + 1, end);
for(TreeNode l: left) {
for(TreeNode r : right) {
TreeNode root = new TreeNode(i);
root.left = l;
root.right = r;
tn.add(root);
}
}
}
memo.put(new StartEnd(start, end), tn);
return tn;
}
public static List<TreeNode> generateTrees(int n) {
return treesStartToEnd(1, n);
}
}

2. Kotlin
class TreeNode(var val: Int) {
var left: TreeNode? = null
var right: TreeNode? = null
}
val memo: MutableMap<Pair<Int, Int>, MutableList<TreeNode?>> = mutableMapOf()
fun treesStartToEnd(start: Int, end: Int): MutableList<TreeNode?> {
val tn: MutableList<TreeNode?> = mutableListOf()
if (start > end) {
tn.add(null)
return tn
}
if (start == end) {
tn.add(TreeNode(start))
return tn
}
if (memo.contains((start to end))) {
return memo[(start to end)]!!
}
for (i in start..end) {
val left = treesStartToEnd(start, i - 1)
val right = treesStartToEnd(i + 1, end)
left.forEach { l ->
right.forEach { r ->
tn.add(
TreeNode(i).also {
it.right = r
it.left = l
}
)
}
}
}
memo[(start to end)] = tn
return tn
}
fun generateTrees(n: Int): List<TreeNode?> {
return treesStartToEnd(1, n)
}

https://github.com/jeongum/algorithm/blob/master/dp/LT_95_UniqueBinarySearchTrees2.kt
'๐ ์๊ณ ๋ฆฌ์ฆ > Dynamic Programming' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[LeetCode] 403. Frog Jump (ํด์, Java ํ์ด) (0) | 2023.08.28 |
---|---|
[์๊ณ ๋ฆฌ์ฆ] Dynamic Programming (0) | 2023.08.05 |